Dexterity II: Talks#
In this part you will solve the following task:
Create a contenttype 'talk' to store all the data required for a talk
Topics covered:
Registration and configuration of content types
Schema
Fields
Widgets
The type registration#
Add a new file types.xml
to your add-on package in profiles/default/
.
That is backend/src/ploneconf.site/sources/ploneconf/site/profiles/default/types.xml
The following lines will tell Plone that there is a new content type defined.
<?xml version="1.0"?>
<object name="portal_types" meta_type="Plone Types Tool">
<object name="talk" meta_type="Dexterity FTI"/>
</object>
Plone will now expect a file profiles/default/types/talk.xml
and will register that as a new content type.
The FTI#
Add the file ploneconf/site/profiles/default/types/talk.xml
.
Note that there is a file types and a folder types.
This is the Factory Type Information that holds the configuration for the content type talk.
1<?xml version="1.0"?>
2<object name="talk" meta_type="Dexterity FTI" i18n:domain="plone"
3 xmlns:i18n="http://xml.zope.org/namespaces/i18n">
4 <property name="title" i18n:translate="">Talk</property>
5 <property name="description" i18n:translate=""></property>
6 <property name="icon_expr">string:${portal_url}/document_icon.png</property>
7 <property name="factory">talk</property>
8 <property name="add_view_expr">string:${folder_url}/++add++talk</property>
9 <property name="link_target"></property>
10 <property name="immediate_view">view</property>
11 <property name="global_allow">True</property>
12 <property name="filter_content_types">True</property>
13 <property name="allowed_content_types"/>
14 <property name="allow_discussion">False</property>
15 <property name="default_view">view</property>
16 <property name="view_methods">
17 <element value="view"/>
18 </property>
19 <property name="default_view_fallback">False</property>
20 <property name="add_permission">cmf.AddPortalContent</property>
21 <property name="klass">ploneconf.site.content.talk.Talk</property>
22 <property name="schema">ploneconf.site.content.talk.ITalk</property>
23 <property name="behaviors">
24 <element value="plone.dublincore"/>
25 <element value="plone.namefromtitle"/>
26 <element value="plone.versioning" />
27 </property>
28 <property name="model_source"></property>
29 <property name="model_file"></property>
30 <property name="schema_policy">dexterity</property>
31 <alias from="(Default)" to="(dynamic view)"/>
32 <alias from="edit" to="@@edit"/>
33 <alias from="sharing" to="@@sharing"/>
34 <alias from="view" to="(selected layout)"/>
35 <action title="View" action_id="view" category="object" condition_expr=""
36 description="" icon_expr="" link_target="" url_expr="string:${object_url}"
37 visible="True">
38 <permission value="View"/>
39 </action>
40 <action title="Edit" action_id="edit" category="object" condition_expr=""
41 description="" icon_expr="" link_target=""
42 url_expr="string:${object_url}/edit" visible="True">
43 <permission value="Modify portal content"/>
44 </action>
45</object>
Now our package has a new configuration for Generic Setup.
Generic Setup loads a lot of different types of configuration for the site from folder profiles/
.
This configuration is applied to your site upon installing the package.
This also means that you will need to re-install the package once we are finished with the talk.
But the type is not yet complete since the schema (ploneconf.site.content.talk.ITalk
) and the class (ploneconf.site.content.talk.Talk
) that are referenced in the FTI are not yet there.
The schema#
The schema holds the definition of the fields that the content type will offer to store data.
It is also the place where you would add widget options per field to control the display of fields.
In the FTI we referenced the Python path ploneconf.site.content.talk.ITalk
.
The module content
does not exist. Create a folder content
and add an empty __init__.py
in it.
From the training root that is backend/src/ploneconf.site/src/ploneconf/site/content/__init__.py
.
In this new folder add a file talk.py
with the following content:
1from plone import schema
2from plone.app.textfield import RichText
3from plone.autoform import directives
4from plone.dexterity.content import Container
5from plone.namedfile.field import NamedBlobImage
6from plone.schema.email import Email
7from plone.supermodel import model
8from z3c.form.browser.checkbox import CheckBoxFieldWidget
9from z3c.form.browser.radio import RadioFieldWidget
10from zope.interface import implementer
11from zope.schema.vocabulary import SimpleTerm
12from zope.schema.vocabulary import SimpleVocabulary
13
14
15class ITalk(model.Schema):
16 """Dexterity-Schema for Talks"""
17
18 directives.widget(type_of_talk=RadioFieldWidget)
19 type_of_talk = schema.Choice(
20 title='Type of talk',
21 values=['Talk', 'Training', 'Keynote'],
22 required=True,
23 )
24
25 details = RichText(
26 title='Details',
27 description='Description of the talk (max. 2000 characters)',
28 max_length=2000,
29 required=True,
30 )
31
32 directives.widget(audience=CheckBoxFieldWidget)
33 audience = schema.Set(
34 title='Audience',
35 value_type=schema.Choice(
36 values=['Beginner', 'Advanced', 'Professional'],
37 ),
38 required=False,
39 )
40
41 speaker = schema.TextLine(
42 title='Speaker',
43 description='Name (or names) of the speaker',
44 required=False,
45 )
46
47 company = schema.TextLine(
48 title='Company',
49 required=False,
50 )
51
52 email = Email(
53 title='Email',
54 description='Email adress of the speaker',
55 required=False,
56 )
57
58 website = schema.TextLine(
59 title='Website',
60 required=False,
61 )
62
63 twitter = schema.TextLine(
64 title='Twitter name',
65 required=False,
66 )
67
68 github = schema.TextLine(
69 title='Github username',
70 required=False,
71 )
72
73 image = NamedBlobImage(
74 title='Image',
75 description='Portrait of the speaker',
76 required=False,
77 )
78
79 speaker_biography = RichText(
80 title='Speaker Biography (max. 1000 characters)',
81 max_length=1000,
82 required=False,
83 )
84
85
86@implementer(ITalk)
87class Talk(Container):
88 """Talk instance class"""
The first class ITalk
is the schema for talks and defines quite a lot of different fields for different kinds of data.
The fields in the schema are mostly from
zope.schema
.The most basic field is
schema.TextLine
which can store text.In the next chapter you will find a reference of all field-types available in Plone.
The widget directives can be ignored by now, as we are implementing for a frontend app. The widget directives like above do control the rendering of the fields in Plone Classic. In the rare case that you need to tweak the rendering of a field in frontend, this can be done like described in plone6docs:volto/recipes/widget
Todo
As a first step use a simplified schema without directives or vocabularies
Then add some simple widget-directives
In the sponsors-chapter discuss all fields, directives, permissions, defaults.
Extend to the final version like collective/ploneconf.site#files in a later chapter
The instance class#
The second class Talk
in talk.py
will be the class of instances for each talk.
It inherits from Container
which is one of the default classes of dexterity.
Container
is used for items that can contain other items.
It does nothing so far but it can be useful later when we want to add methods or properties to it that can be used directly from a talk instance.
Try the new type#
Now all pieces should be in place and you can enable the new type Talk
.
Restart Plone (to load the new Python code and the changed zcml)
You do not need to restart the Volto frontend since we did not do any changes there.
Re-install the package ploneconf.site (deactivate and activate) to load the type registration and type configuration: Follow the link "SITE SETUP" in the bottom of the toolbar and switch to "Add-Ons".
Now instances of the new type can be added. Please check that you can add a talk to your site.
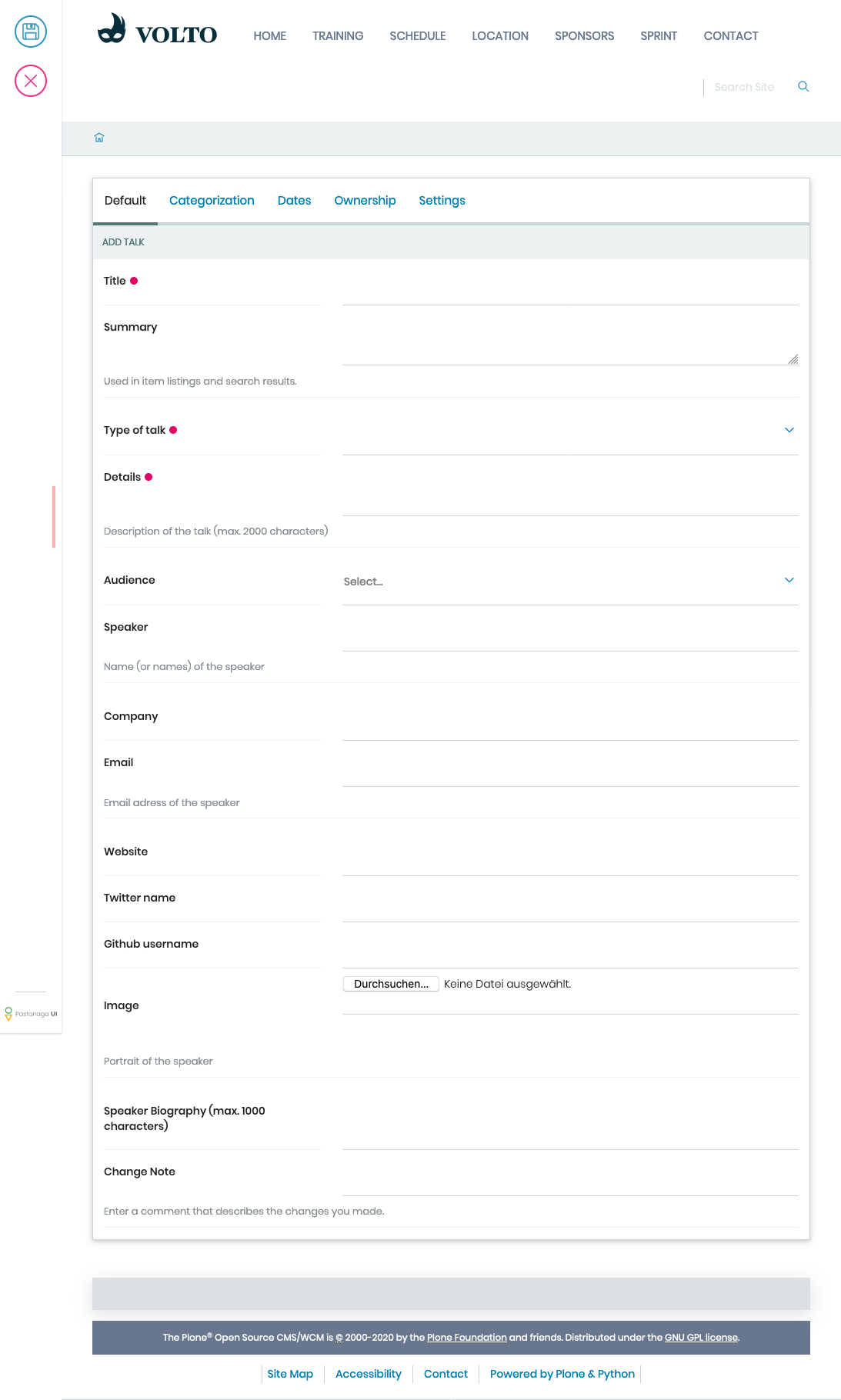
Adding a talk in the frontend#
Test the type by adding a talk. Add some values in the fields, save it, look at the view and edit it again.
Compare all the fields you see to the code in the schema.
You can also make changes in the schema. After restarting the backend these changes are effective immediately.
Find the tool
portal_types
in the ZMI http://localhost:8080/manage. Look at the FTI for typetalk
and inspect the configuration taken from the FTI.You can make changes to the FTI here.
A part of the configuration is also available in Plone control panels (SITE SETUP). For example the content types control panel
http://localhost:3000/controlpanel/dexterity-types
allows to add behaviors to content types. Please be aware that these changes are done on your site instance. You can use the browser UI, but it's the add-on package where you configure your content types.
The field values of your talk instance are listed. In one of the next chapters we will create a custom view for the new type.
Summary#
You created a custom content type.
You can now control the data that will be stored for talks.
You can reuse and adapt these examples to model data for your own use-cases.
Next up: After looking at even more fields that are available in Plone, you will learn to change how talks are displayed.